Step 1
Make a new Android Project named Camera App
Step 2
Include the following permissions in Manifest.xml file :
Step 3
Make the Layout File activity_main.xml:
Step 4
MainActivity.java file
Initialize the request, the constants and view:
private static final int IMAGE_REQUEST_CODE=1;
private static final String IMAGE_DIRECTORY_NAME = “Hello Camera”;
private Uri fileUri;
private ImageView img;
private Button clk= (Button) findViewById(R.id.button1);
Method to capture the Image:
private void captureImage() {
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
fileUri = getOutputMediaFileUri(MEDIA_TYPE_IMAGE);
intent.putExtra(MediaStore.EXTRA_OUTPUT, fileUri);
startActivityForResult(intent, IMAGE_REQUEST_CODE);
}
Method OnActivityResult:
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
// if the result is capturing Image
if (requestCode == CAMERA_CAPTURE_IMAGE_REQUEST_CODE) {
if (resultCode == RESULT_OK) {
previewCapturedImage();
} else if (resultCode == RESULT_CANCELED) {
Toast.makeText(getApplicationContext(),
“User cancelled image capture”, Toast.LENGTH_SHORT)
.show();
} else {
// failed to capture image
Toast.makeText(getApplicationContext(),
“Sorry! Failed to capture image”, Toast.LENGTH_SHORT)
.show();
}
}
}
Display Picture
BitmapFactory is a class for images which is used to display the picture on the screen.
private void previewCapturedImage() {
// bimatp factory
BitmapFactory.Options options = new BitmapFactory.Options();
options.inSampleSize = 8;
final Bitmap bitmap = BitmapFactory.decodeFile(fileUri.getPath(),
options);
imgPreview.setImageBitmap(bitmap);
}
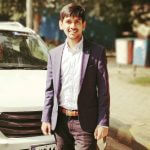
strategies your digital product..